11/08/2018, 19:24
MyBatis Example – mapper bằng Annotations
MyBatis là gì? Các công nghệ được sử dụng trong ví dụ này: Eclipse KEPLER SR2 MySQL 5.0.11 MyBatis 3.3.0 JDK 1.8 Cấu trúc project Các bước thực hiện 1. Tạo project “mybatis-annotation-example-1” Các bạn tạo project có tên ...
MyBatis là gì?
Các công nghệ được sử dụng trong ví dụ này:
- Eclipse KEPLER SR2
- MySQL 5.0.11
- MyBatis 3.3.0
- JDK 1.8
Cấu trúc project
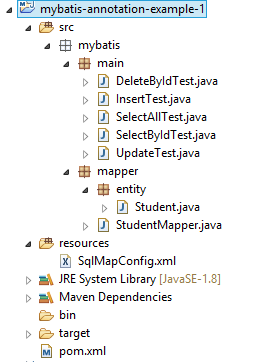
Các bước thực hiện
1. Tạo project “mybatis-annotation-example-1”
Các bạn tạo project có tên “mybatis-annotation-example-1” như trong bài hướng dẫn Tạo MyBatis project trong eclipse
2. Tạo bảng details.student
CREATE TABLE details.student( ID int(10) NOT NULL AUTO_INCREMENT, NAME varchar(100) NOT NULL, BRANCH varchar(255) NOT NULL, PERCENTAGE int(3) NOT NULL, PHONE int(10) NOT NULL, EMAIL varchar(255) NOT NULL, PRIMARY KEY ( ID ) );
3. Tạo lớp Student trong package mybatis.mapper.entity
File: Student.java
package mybatis.mapper.entity; public class Student { private int id; private String name; private String branch; private int percentage; private int phone; private String email; public Student() { } public Student(String name, String branch, int percentage, int phone, String email) { super(); this.name = name; this.branch = branch; this.percentage = percentage; this.phone = phone; this.email = email; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getBranch() { return branch; } public void setBranch(String branch) { this.branch = branch; } public int getPercentage() { return percentage; } public void setPercentage(int percentage) { this.percentage = percentage; } public int getPhone() { return phone; } public void setPhone(int phone) { this.phone = phone; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } @Override public String toString() { return getClass().getName() + "[" + "id = " + id + ", name = " + name + ", branch = " + branch + ", percentage = " + percentage + ", phone = " + phone + ", email = " + email + "]"; } }
4. Tạo file cấu hình MyBatis SqlMapConfig.xml trong resources
File: SqlMapConfig.xml
<?xml version = "1.0" encoding = "UTF-8"?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <environments default="development"> <environment id="development"> <transactionManager type="JDBC" /> <dataSource type="POOLED"> <property name="driver" value="com.mysql.jdbc.Driver" /> <property name="url" value="jdbc:mysql://localhost:3306/details" /> <property name="username" value="root" /> <property name="password" value="1234567890" /> </dataSource> </environment> </environments> <mappers> <mapper class="mybatis.mapper.StudentMapper" /> </mappers> </configuration>
5. Tạo interface StudentMapper
Tạo interface StudentMapper để định nghĩa các câu lệnh mapped SQL.
File: StudentMapper.java
package mybatis.mapper; import java.util.List; import org.apache.ibatis.annotations.Delete; import org.apache.ibatis.annotations.Insert; import org.apache.ibatis.annotations.Options; import org.apache.ibatis.annotations.Result; import org.apache.ibatis.annotations.Results; import org.apache.ibatis.annotations.Select; import org.apache.ibatis.annotations.Update; import mybatis.mapper.entity.Student; public interface StudentMapper { // get all student final String GET_ALL_STUDENT = "SELECT * FROM STUDENT"; @Select(GET_ALL_STUDENT) @Results(value = { @Result(property = "id", column = "ID"), @Result(property = "name", column = "NAME"), @Result(property = "branch", column = "BRANCH"), @Result(property = "percentage", column = "PERCENTAGE"), @Result(property = "phone", column = "PHONE"), @Result(property = "email", column = "EMAIL") }) public List<Student> getAll(); // get student by id final String GET_STUDENT_BY_ID = "SELECT * FROM STUDENT WHERE ID = #{id}"; @Select(GET_STUDENT_BY_ID) public Student getById(int id); // inert student final String INSERT_STUDENT = "INSERT INTO STUDENT (NAME, BRANCH, PERCENTAGE, PHONE, EMAIL ) " + "VALUES (#{name}, #{branch}, #{percentage}, #{phone}, #{email})"; @Update(INSERT_STUDENT) @Options(useGeneratedKeys = true, keyProperty = "id") public void insert(Student student); // update student final String UPDATE_STUDENT = "UPDATE STUDENT SET EMAIL = #{email}, NAME = #{name}, " + "BRANCH = #{branch}, PERCENTAGE = #{percentage}, PHONE = #{phone} WHERE ID = #{id}"; @Insert(UPDATE_STUDENT) public void update(Student student); // delete student by id final String DELETE_STUDENT_BY_ID = "DELETE from STUDENT WHERE ID = #{id}"; @Delete(DELETE_STUDENT_BY_ID) public void delete(int id); }
6. Tạo các class để test
File: SelectAllTest.java
package mybatis.main; import java.io.IOException; import java.io.Reader; import java.util.List; import org.apache.ibatis.io.Resources; import org.apache.ibatis.session.SqlSession; import org.apache.ibatis.session.SqlSessionFactory; import org.apache.ibatis.session.SqlSessionFactoryBuilder; import mybatis.mapper.StudentMapper; import mybatis.mapper.entity.Student; public class SelectAllTest { public static void main(String[] args) throws IOException { Reader reader = Resources.getResourceAsReader("SqlMapConfig.xml"); SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(reader); SqlSession session = sqlSessionFactory.openSession(); // create student mapper StudentMapper studentMapper = session.getMapper(StudentMapper.class); // show list student List<Student> listStudents = studentMapper.getAll(); for (Student student : listStudents) { System.out.println(student.toString()); } // close session session.close(); } }
File: SelectByIdTest.java
public class SelectByIdTest { public static void main(String[] args) throws IOException { Reader reader = Resources.getResourceAsReader("SqlMapConfig.xml"); SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(reader); SqlSession session = sqlSessionFactory.openSession(); // create student mapper StudentMapper studentMapper = session.getMapper(StudentMapper.class); // get student by Id Student student = studentMapper.getById(12); System.out.println(student); // close session session.close(); } }
File: InsertTest.java
public class InsertTest { public static void main(String[] args) throws IOException { Reader reader = Resources.getResourceAsReader("SqlMapConfig.xml"); SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(reader); SqlSession session = sqlSessionFactory.openSession(); // create student mapper StudentMapper studentMapper = session.getMapper(StudentMapper.class); // insert student Student student = new Student("Jim", "IT", 90, 1234567, "jim-it@gmail.com"); studentMapper.insert(student); session.commit(); System.out.println("insert sucessfully"); // close session session.close(); } }
File: UpdateTest.java
public class UpdateTest { public static void main(String[] args) throws IOException { Reader reader = Resources.getResourceAsReader("SqlMapConfig.xml"); SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(reader); SqlSession session = sqlSessionFactory.openSession(); // create student mapper StudentMapper studentMapper = session.getMapper(StudentMapper.class); // update student Student student = studentMapper.getById(12); student.setName("Jim Smith"); student.setPhone(12399888); studentMapper.update(student); session.commit(); System.out.println("update sucessfully"); // close session session.close(); } }
File: DeleteByIdTest.java
public class DeleteByIdTest { public static void main(String[] args) throws IOException { Reader reader = Resources.getResourceAsReader("SqlMapConfig.xml"); SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(reader); SqlSession session = sqlSessionFactory.openSession(); // create student mapper StudentMapper studentMapper = session.getMapper(StudentMapper.class); // delete student studentMapper.delete(11); session.commit(); System.out.println("delete successfully"); // close session session.close(); } }
Download Source Code
Download Now!
MyBatis là gì?