Ví dụ về Hibernate
Session trong Hibernate Các công nghệ sử dụng trong ví dụ này eclipse-jee-mars-2 MySQL 5.0.11 JDK 1.8 hibernate 3.6.3 Cấu trúc project Các bước thực hiện 1. Tạo project hibernate-example Tạo project “hibernate-example” tham ...
Các công nghệ sử dụng trong ví dụ này
- eclipse-jee-mars-2
- MySQL 5.0.11
- JDK 1.8
- hibernate 3.6.3
Cấu trúc project
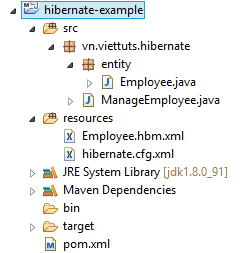
Các bước thực hiện
1. Tạo project hibernate-example
Tạo project “hibernate-example” tham khảo các bước như trong bài hướng dẫn Tạo MyBatis project trong eclipse
Add các dependency vào file pom.xml
<dependencies> <dependency> <groupId>org.hibernate</groupId> <artifactId>hibernate-core</artifactId> <version>3.6.3.Final</version> </dependency> <dependency> <groupId>javassist</groupId> <artifactId>javassist</artifactId> <version>3.12.1.GA</version> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>5.1.6</version> </dependency> </dependencies>
2. Tạo lớp POJO
Bước đầu tiên trong việc tạo một ứng dụng là xây các lớp Java POJO, tùy thuộc vào ứng dụng persisted với cơ sở dữ liệu. Tạo lớp Employee các phương pháp getXXX và setXXX để làm cho nó phù hợp với lớp JavaBeans.
Một POJO (Plain Old Java Object) là một đối tượng Java không extends hoặc implements một số lớp và interface chuyên biệt tương ứng theo yêu cầu của EJB Framework. Tất cả các đối tượng Java thông thường là POJO.
Khi bạn thiết kế một lớp để persisted Hibernate, điều quan trọng là phải viết code tuân thủ JavaBeans cũng như một thuộc tính sẽ hoạt động như một chỉ mục như thuộc tính id trong lớp Employee.
public class Employee { private int id; private String firstName; private String lastName; private int salary; public Employee() {} public Employee(String fname, String lname, int salary) { this.firstName = fname; this.lastName = lname; this.salary = salary; } public int getId() { return id; } public void setId( int id ) { this.id = id; } public String getFirstName() { return firstName; } public void setFirstName( String first_name ) { this.firstName = first_name; } public String getLastName() { return lastName; } public void setLastName( String last_name ) { this.lastName = last_name; } public int getSalary() { return salary; } public void setSalary( int salary ) { this.salary = salary; } }
3. Tạo các bảng trong cơ sở dữ liệu
Cần phải tạo các bảng tương ứng với mỗi đối tượng persistent. Các đối tượng trên cần phải được lưu trữ và truy xuất vào bảng RDBMS sau:
create table testdb.EMPLOYEE ( id INT NOT NULL auto_increment, first_name VARCHAR(20) default NULL, last_name VARCHAR(20) default NULL, salary INT default NULL, PRIMARY KEY (id) );
4. Tạo file mapping
Bước này là tạo ra một file mapping để hướng dẫn Hibernate làm thế nào để ánh xạ lớp POJO java vào các bảng cơ sở dữ liệu.
<?xml version="1.0" encoding="utf-8"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD//EN" "http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd"> <hibernate-mapping> <class name="vn.viettuts.hibernate.entity.Employee" table="EMPLOYEE"> <meta attribute="class-description"> Lớp này chưa thông tin chi tiết về employee. </meta> <id name="id" type="int" column="id"> <generator class="native" /> </id> <property name="firstName" column="first_name" type="string" /> <property name="lastName" column="last_name" type="string" /> <property name="salary" column="salary" type="int" /> </class> </hibernate-mapping>
5. Tạo file cấu hình Hibernate
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE hibernate-configuration SYSTEM "http://www.hibernate.org/dtd/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <property name="hibernate.dialect"> org.hibernate.dialect.MySQLDialect </property> <property name="hibernate.connection.driver_class"> com.mysql.jdbc.Driver </property> <property name="hibernate.connection.url"> jdbc:mysql://localhost/testdb </property> <property name="hibernate.connection.username"> root </property> <property name="hibernate.connection.password"> 1234567890 </property> <mapping resource="Employee.hbm.xml" /> </session-factory> </hibernate-configuration>
6. Tạo lớp ứng dụng
Cuối cùng, chúng ta sẽ tạo lớp ứng dụng để chạy ứng dụng phương thức main (). Trong lớp này có các method để thực hiện các thao tác CRUD (create, retrive, update, delete).
import java.util.Iterator; import java.util.List; import org.hibernate.HibernateException; import org.hibernate.Session; import org.hibernate.SessionFactory; import org.hibernate.Transaction; import org.hibernate.cfg.Configuration; import vn.viettuts.hibernate.entity.Employee; public class ManageEmployee { private static SessionFactory factory; public static void main(String[] args) { try { factory = new Configuration().configure().buildSessionFactory(); } catch (Throwable ex) { ex.printStackTrace(); } ManageEmployee ME = new ManageEmployee(); // Add few employee records in database Integer empID1 = ME.addEmployee("Zara", "Ali", 1000); Integer empID2 = ME.addEmployee("Daisy", "Das", 5000); Integer empID3 = ME.addEmployee("John", "Paul", 10000); // List down all the employees ME.listEmployees(); // Update employee's records ME.updateEmployee(empID1, 5000); // Delete an employee from the database ME.deleteEmployee(empID2); // List down new list of the employees ME.listEmployees(); } // Method to CREATE an employee in the database public Integer addEmployee(String fname, String lname, int salary) { Session session = factory.openSession(); Transaction tx = null; Integer employeeID = null; try { tx = session.beginTransaction(); Employee employee = new Employee(fname, lname, salary); employeeID = (Integer) session.save(employee); tx.commit(); } catch (HibernateException e) { if (tx != null) tx.rollback(); e.printStackTrace(); } finally { session.close(); } return employeeID; } // Method to READ all the employees public void listEmployees() { Session session = factory.openSession(); Transaction tx = null; try { tx = session.beginTransaction(); List employees = session.createQuery("FROM Employee").list(); for (Iterator iterator = employees.iterator(); iterator.hasNext();) { Employee employee = (Employee) iterator.next(); System.out.print("First Name: " + employee.getFirstName()); System.out.print(" Last Name: " + employee.getLastName()); System.out.println(" Salary: " + employee.getSalary()); } tx.commit(); } catch (HibernateException e) { if (tx != null) tx.rollback(); e.printStackTrace(); } finally { session.close(); } } // Method to UPDATE salary for an employee public void updateEmployee(Integer EmployeeID, int salary) { Session session = factory.openSession(); Transaction tx = null; try { tx = session.beginTransaction(); Employee employee = (Employee) session.get(Employee.class, EmployeeID); employee.setSalary(salary); session.update(employee); tx.commit(); } catch (HibernateException e) { if (tx != null) tx.rollback(); e.printStackTrace(); } finally { session.close(); } } // Method to DELETE an employee from the records public void deleteEmployee(Integer EmployeeID) { Session session = factory.openSession(); Transaction tx = null; try { tx = session.beginTransaction(); Employee employee = (Employee) session.get(Employee.class, EmployeeID); session.delete(employee); tx.commit(); } catch (HibernateException e) { if (tx != null) tx.rollback(); e.printStackTrace(); } finally { session.close(); } } }
Kết quả
Output:
First Name: Zara Last Name: Ali Salary: 1000 First Name: Daisy Last Name: Das Salary: 5000 First Name: John Last Name: Paul Salary: 10000 First Name: Zara Last Name: Ali Salary: 5000 First Name: John Last Name: Paul Salary: 10000
Download Source Code