MyBatis Example – mapper bằng file XML
MyBatis là gì? Các công nghệ được sử dụng trong ví dụ này: Eclipse KEPLER SR2 MySQL 5.0.11 JDK 1.8 MyBatis 3.3.0 Cấu trúc project Các bước thực hiện 1. Tạo project “mybatis-example-1” Các bạn tạo project có tên ...
Các công nghệ được sử dụng trong ví dụ này:
- Eclipse KEPLER SR2
- MySQL 5.0.11
- JDK 1.8
- MyBatis 3.3.0
Cấu trúc project
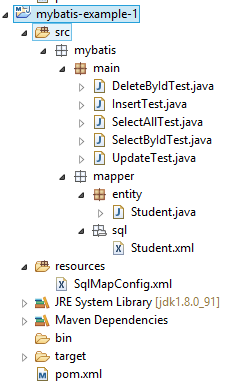
Các bước thực hiện
1. Tạo project “mybatis-example-1”
Các bạn tạo project có tên “mybatis-example-1” như trong bài hướng dẫn Tạo MyBatis project trong eclipse
2. Tạo bảng details.student
CREATE TABLE details.student( ID int(10) NOT NULL AUTO_INCREMENT, NAME varchar(100) NOT NULL, BRANCH varchar(255) NOT NULL, PERCENTAGE int(3) NOT NULL, PHONE int(10) NOT NULL, EMAIL varchar(255) NOT NULL, PRIMARY KEY ( ID ) );
3. Tạo lớp Student trong package mybatis.mapper.entity
File: Student.java
package mybatis.mapper.entity; public class Student { private int id; private String name; private String branch; private int percentage; private int phone; private String email; public Student() { } public Student(String name, String branch, int percentage, int phone, String email) { super(); this.name = name; this.branch = branch; this.percentage = percentage; this.phone = phone; this.email = email; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getBranch() { return branch; } public void setBranch(String branch) { this.branch = branch; } public int getPercentage() { return percentage; } public void setPercentage(int percentage) { this.percentage = percentage; } public int getPhone() { return phone; } public void setPhone(int phone) { this.phone = phone; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } @Override public String toString() { return getClass().getName() + "[" + "id = " + id + ", name = " + name + ", branch = " + branch + ", percentage = " + percentage + ", phone = " + phone + ", email = " + email + "]"; } }
4. Tạo file cấu hình MyBatis SqlMapConfig.xml trong resources
Trong file cấu hình này có sử dụng thẻ typeAliases tạo alias Student có type là lớp mybatis.mapper.entity.Student. Bằng cách này trong các file cấu hình này hoặc trong các file mapper có thể sử dụng tên tắt Student thay vì mybatis.mapper.entity.Student.
File: SqlMapConfig.xml
<?xml version = "1.0" encoding = "UTF-8"?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <typeAliases> <typeAlias alias="Student" type="mybatis.mapper.entity.Student"/> </typeAliases> <environments default="development"> <environment id="development"> <transactionManager type="JDBC" /> <dataSource type="POOLED"> <property name="driver" value="com.mysql.jdbc.Driver" /> <property name="url" value="jdbc:mysql://localhost:3306/details" /> <property name="username" value="root" /> <property name="password" value="1234567890" /> </dataSource> </environment> </environments> <mappers> <mapper resource="mybatis/mapper/sql/Student.xml" /> </mappers> </configuration>
5. Tạo file mapper Student.xml
Đối với kết quả trả về của câu lệnh truy vấn select các bạn có thể dùng thuộc tính resultMap hoặc resultType như được khai báo dưới đây.
File: Student.xml
<?xml version = "1.0" encoding = "UTF-8"?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="Student"> <insert id="insertStudent" parameterType="Student" > INSERT INTO STUDENT (ID, NAME, BRANCH, PERCENTAGE, PHONE, EMAIL ) VALUES (#{id}, #{name}, #{branch}, #{percentage}, #{phone}, #{email}); </insert> <update id="updateStudent" parameterType="Student"> UPDATE STUDENT SET EMAIL = #{email}, NAME = #{name}, BRANCH = #{branch}, PERCENTAGE = #{percentage}, PHONE = #{phone} WHERE ID = #{id}; </update> <delete id="deleteStudentById" parameterType="int"> DELETE from STUDENT WHERE ID = #{id}; </delete> <select id="selectAllStudent" resultMap="result"> SELECT * FROM STUDENT; </select> <select id="selectStudentById" parameterType="int" resultType="Student"> SELECT * FROM STUDENT WHERE ID = #{id}; </select> <resultMap id = "result" type = "Student"> <result property = "id" column = "ID"/> <result property = "name" column = "NAME"/> <result property = "branch" column = "BRANCH"/> <result property = "percentage" column = "PERCENTAGE"/> <result property = "phone" column = "PHONE"/> <result property = "email" column = "EMAIL"/> </resultMap> </mapper>
6. Tạo các class để test
File: SelectAllTest.java
package mybatis.main; import java.io.IOException; import java.io.Reader; import java.util.List; import org.apache.ibatis.io.Resources; import org.apache.ibatis.session.SqlSession; import org.apache.ibatis.session.SqlSessionFactory; import org.apache.ibatis.session.SqlSessionFactoryBuilder; import mybatis.mapper.entity.Student; public class SelectAllTest { public static void main(String[] args) throws IOException { // read config file Reader reader = Resources.getResourceAsReader("SqlMapConfig.xml"); SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(reader); // open session SqlSession session = sqlSessionFactory.openSession(); // get all student List<Student> listStudents = session.selectList("Student.selectAllStudent"); for (Student student : listStudents) { System.out.println(student); } // close session session.close(); } }
File: SelectByIdTest.java
public class SelectByIdTest { public static void main(String[] args) throws IOException { // read config file Reader reader = Resources.getResourceAsReader("SqlMapConfig.xml"); SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(reader); // open session SqlSession session = sqlSessionFactory.openSession(); // get student by Id Student student = session.selectOne("Student.selectStudentById", 10); System.out.println(student); // close session session.close(); } }
File: InsertTest.java
public class InsertTest { public static void main(String[] args) throws IOException { // read config file Reader reader = Resources.getResourceAsReader("SqlMapConfig.xml"); SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(reader); // open session SqlSession session = sqlSessionFactory.openSession(); // insert student Student student = new Student("Jim", "IT", 100, 123999, "jim-it@gmail.com"); session.insert("Student.insertStudent", student); session.commit(); // close session session.close(); } }
File: UpdateTest.java
public class UpdateTest { public static void main(String[] args) throws IOException { // read config file Reader reader = Resources.getResourceAsReader("SqlMapConfig.xml"); SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(reader); // open session SqlSession session = sqlSessionFactory.openSession(); // update student Student student = session.selectOne("Student.selectStudentById", 10); student.setName("Jim Smith"); student.setPhone(12399888); session.update("Student.update", student); session.commit(); // close session session.close(); } }
File: DeleteByIdTest.java
public class DeleteByIdTest { public static void main(String[] args) throws IOException { // read config file Reader reader = Resources.getResourceAsReader("SqlMapConfig.xml"); SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(reader); // open session SqlSession session = sqlSessionFactory.openSession(); // delete student session.delete("Student.deleteStudentById", 10); session.commit(); // close session session.close(); } }
Download Source Code