Kế thừa Bean trong Spring
Previous Trong một định nghĩa bean có thể chứa rất nhiều thông tin cấu hình, bao gồm các đối số constructor, các giá trị thuộc tính và thông tin cụ thể của vùng chứa như phương thức khởi tạo, tên phương thức static factory, v.v. Kế thừa Bean trong Spring , định nghĩa bean con ...
Trong một định nghĩa bean có thể chứa rất nhiều thông tin cấu hình, bao gồm các đối số constructor, các giá trị thuộc tính và thông tin cụ thể của vùng chứa như phương thức khởi tạo, tên phương thức static factory, v.v.
Kế thừa Bean trong Spring, định nghĩa bean con thừa kế dữ liệu cấu hình từ định nghĩa bean cha. Định nghĩa con có thể ghi đè lên một số giá trị hoặc thêm các giá trị khác nếu cần.
Định nghĩa kế thừa Bean trong Spring không liên quan gì đến việc kế thừa lớp Java nhưng khái niệm kế thừa là giống nhau. Bạn có thể định nghĩa một định nghĩa bean cha làm mẫu và các bean con khác có thể kế thừa các cấu hình cần thiết từ bean cha.
Khi bạn sử dụng siêu dữ liệu cấu hình dựa trên XML, bạn chỉ định định nghĩa bean con bằng cách sử dụng thuộc tính parent, chỉ định tên của bean cha làm giá trị của thuộc tính này.
Ví dụ kế thừa Bean trong Spring
Tạo một Java Maven project trong Eclipse.
Cấu trúc project:
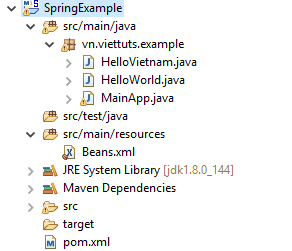
Sau đó update file pom.xml để tải thư viện Spring như sau:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>vn.viettuts</groupId> <artifactId>SpringExample</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>SpringExample</name> <url>http://maven.apache.org</url> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <dependencies> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>4.3.6.RELEASE</version> <scope>provided</scope> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-beans</artifactId> <version>4.3.6.RELEASE</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>4.3.6.RELEASE</version> </dependency> </dependencies> </project>
Sau đây là file cấu hình Beans.xml nơi chúng ta định nghĩa bean “helloWorld” có hai thuộc tính message1 và message2. Tiếp theo là bean “helloVietnam” được định nghĩa là con của bean “helloWorld” bằng cách sử dụng thuộc tính parent. Bean con thừa hưởng thuộc tính message2 và ghi đè thuộc tính message1 và có thêm một thuộc tính message3.
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd"> <bean id="helloWorld" class="vn.viettuts.example.HelloWorld"> <property name="message1" value="Hello World!" /> <property name="message2" value="Hello Second World!" /> </bean> <bean id=" helloVietnam" class="vn.viettuts.example.HelloVietnam" parent="helloWorld"> <property name="message1" value="Hello Vietnam" /> <property name="message3" value="Welcome to Vietnam!" /> </bean> </beans>
Đây là nội dung của lớp HelloWorld.java
package vn.viettuts.example; public class HelloWorld { private String message1; private String message2; public void setMessage1(String message1) { this.message1 = message1; } public void getMessage1() { System.out.println("Your Message : " + message1); } public void setMessage2(String message2) { this.message2 = message2; } public void getMessage2() { System.out.println("Your Message : " + message2); } }
Đây là nội dung của lớp HelloVietnam.java
package vn.viettuts.example; public class HelloVietnam { private String message1; private String message2; private String message3; public void setMessage1(String message1) { this.message1 = message1; } public void getMessage1() { System.out.println("Your Message : " + message1); } public void setMessage2(String message2) { this.message2 = message2; } public void getMessage2() { System.out.println("Your Message : " + message2); } public void setMessage3(String message3) { this.message3 = message3; } public void getMessage3() { System.out.println("Your Message : " + message3); } }
Đây là nội dung của lớp MainApp.java
package vn.viettuts.example; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; /** * Hello world! * */ public class MainApp { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext( "Beans.xml"); HelloWorld objA = (HelloWorld) context.getBean("helloWorld"); objA.getMessage1(); objA.getMessage2(); HelloVietnam objB = (HelloVietnam) context.getBean("helloVietnam"); objB.getMessage1(); objB.getMessage2(); objB.getMessage3(); } }
Kết quả:
Your Message : Hello World! Your Message : Hello Second World! Your Message : Hello India! Your Message : Hello Second World! Your Message : Namaste India!
Chúng ta đã không truyền giá trị cho thuộc tính message2 trong khi tạo bean “helloVietnam”, nhưng nó đã được truyền giá trị vì kế thừa bean “helloWorld”.
Mẫu định nghĩa Bean
Bạn có thể tạo một mẫu định nghĩa Bean, có thể được sử dụng bởi các Bean con khác. Trong khi xác định nghĩa mẫu Định nghĩa Bean, bạn không nên chỉ định thuộc tính class và nên chỉ định thuộc tính abstract và phải chỉ định thuộc tính abstract có giá trị “true” như được hiển thị trong ví dụ sau:
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd"> <bean id="beanTeamplate" abstract="true"> <property name="message1" value="Hello World!" /> <property name="message2" value="Hello Second World!" /> <property name="message3" value="Welcome to Vietnam!" /> </bean> <bean id=" helloVietnam" class="vn.viettuts.example.HelloVietnam" parent="beanTeamplate"> <property name="message1" value="Hello Vietnam" /> <property name="message3" value="Welcome to Vietnam!" /> </bean> </beans>
Bean cha không thể tự khởi tạo được vì nó không liên kết đến một lớp cụ thể, và nó cũng được đánh dấu rõ ràng là trừu tượng (abstract = “true”). Khi đó, nó chỉ có thể sử dụng như một định nghĩa bean mẫu được kế thừa bởi các Bean con.