Service trong Angular7
Routing trong Angular 7 Nội dung chính Giới thiệu Service trong Angular Tạo service trong Angular Khai báo service trong module app.module.ts Tạo hàm cho service trong Angular Sử dụng hàm showTodayDate() trong component new-cmp.component.ts Sử dụng ...
Nội dung chính
- Giới thiệu Service trong Angular
- Tạo service trong Angular
- Khai báo service trong module app.module.ts
-
Tạo hàm cho service trong Angular
- Sử dụng hàm showTodayDate() trong component new-cmp.component.ts
- Sử dụng hàm showTodayDate() trong component app.component.ts
- Kết quả
- Thay đổi thuộc tính của service
Giới thiệu Service trong Angular
Chúng ta có thể gặp một tình huống mà chúng ta cần một code để sử dụng ở mọi nơi trên trang. Chẳng hạn như kết nối dữ liệu cần được chia sẻ giữa các component. Với Service, chúng ta có thể truy cập các phương thức và thuộc tính trên các component khác trong toàn bộ dự án.
Tạo service trong Angular
Để tạo một service, chúng ta cần sử dụng dòng lệnh như dưới đây:
ng g service myservice
Kết quả:
C:AngularAngular7my-app>ng g service myservice CREATE src/app/myservice.service.spec.ts (348 bytes) CREATE src/app/myservice.service.ts (138 bytes)
Các file được tạo ra trong thư mục ứng dụng như sau:
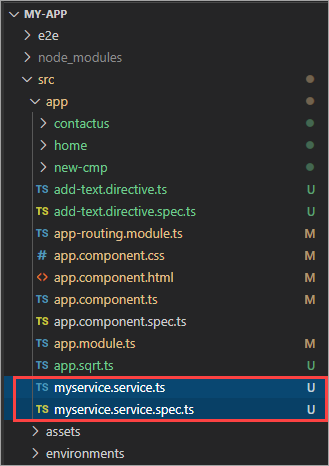
Sau đây là các file đã tạo myservice.service.specs.ts và myservice.service.ts.
myservice.service.ts
import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root' }) export class MyserviceService { constructor() { } }
Ở đây, mô-đun Injectable được import từ @angular/core. Nó chứa phương thức @Injectable và một lớp gọi là MyserviceService. Chúng ta sẽ tạo chức năng service trong lớp này.
Khai báo service trong module app.module.ts
Để sử dụng service, cũng giống như component, chúng ta cần phải khai báo service trong module chính app.module.ts
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AppRoutingModule, RoutingComponent } from './app-routing.module'; import { AppComponent } from './app.component'; import { NewCmpComponent } from './new-cmp/new-cmp.component'; import { AddTextDirective } from './add-text.directive'; import { SqrtPipe, SquarePipe } from './app.sqrt'; import { HomeComponent } from './home/home.component'; import { ContactusComponent } from './contactus/contactus.component'; import { MyserviceService } from './myservice.service'; @NgModule({ declarations: [ AppComponent, NewCmpComponent, AddTextDirective, SqrtPipe, SquarePipe, HomeComponent, ContactusComponent, RoutingComponent ], imports: [ BrowserModule, AppRoutingModule ], providers: [MyserviceService], bootstrap: [AppComponent] }) export class AppModule { }
Trong file trên, chúng ta đã nhập Service với tên lớp và lớp tương tự được khai báo trong mảng providers (providers: [MyserviceService]).
Tạo hàm cho service trong Angular
Bây giờ chúng ta sẽ chuyển trở lại lớp MyserviceService và tạo một chức năng trong nó.
Trong lớp MyserviceService, chúng ta sẽ tạo một hàm hiển thị ngày hôm nay. Sau đó sử dụng một hàm này trong app.component.ts và trong new-cmp.component.ts mà chúng ta đã tạo trong bài trước.
Tạo hàm: showTodayDate()
import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root' }) export class MyserviceService { constructor() { } showTodayDate() { let today = new Date(); return today; } }
Sử dụng hàm showTodayDate() trong component new-cmp.component.ts
Để sử dụng hàm showTodayDate() trong component new-cmp.component.ts, đầu tiên chúng ta cần phải inject lớp MyServiceService vào constructor của lớp AppComponent trong file new-cmp.component.ts. Sau đó chúng ta có thể gọi hàm showTodayDate() như sau:
new-cmp.component.ts
import { Component, OnInit } from '@angular/core'; import { MyserviceService } from '../myservice.service'; @Component({ selector: 'app-new-cmp', templateUrl: './new-cmp.component.html', styleUrls: ['./new-cmp.component.css'] }) export class NewCmpComponent implements OnInit { newcomponent = "New Component"; todayDate: Date; constructor(private myservice: MyserviceService) { } ngOnInit() { this.todayDate = this.myservice.showTodayDate(); } }
Hiển thị biến todayDate trong view của component new-cmp.component.ts
new-cmp.component.html
<p> {{newcomponent}} </p> <p> Today's Date : {{todayDate}} </p>
Sử dụng hàm showTodayDate() trong component app.component.ts
Để sử dụng hàm showTodayDate() trong component app.component.ts, đầu tiên chúng ta cần phải inject lớp MyServiceService vào constructor của lớp AppComponent trong file app.component.ts. Sau đó chúng ta có thể gọi hàm showTodayDate() như sau:
app.component.ts
import { Component } from '@angular/core'; import { MyserviceService } from './myservice.service'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 7 Project!'; todayDate: Date; constructor(private myservice: MyserviceService) {} ngOnInit() { this.todayDate = this.myservice.showTodayDate(); } }
Hiển thị biến todayDate trong view của component app.component.ts, và hiển thị nội dung của new-cmp.component.html:
app.component.html
<h2> App Component </h2> {{todayDate}} <app-new-cmp></app-new-cmp>
Kết quả
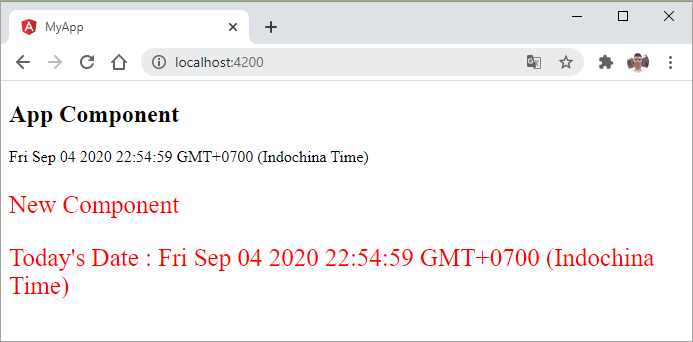
Thay đổi thuộc tính của service
Nếu bạn thay đổi thuộc tính của service trong bất kỳ component nào, thì các component khác cũng thay đổi như vậy. Bây giờ chúng ta cùng tìm hiểu cách này hoạt động này qua ví dụ sau.
Tạo biến và gán giá trị serviceproperty = "Service Created" cho lớp service MyserviceService:
myservice.service.ts
import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root' }) export class MyserviceService { // khoi tao bien serviceproperty serviceproperty = "Service Created"; constructor() { } showTodayDate() { let today = new Date(); return today; } }
Thay đổi giá trị của biến MyserviceService.serviceproperty trong lớp AppComponent và gán nó cho biến AppComponent.property .
app.component.ts
import { Component } from '@angular/core'; import { MyserviceService } from './myservice.service'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 7 Project!'; todayDate: Date; property: string; constructor(private myservice: MyserviceService) {} ngOnInit() { this.todayDate = this.myservice.showTodayDate(); this.myservice.serviceproperty = "Change property in AppComponent." this.property = this.myservice.serviceproperty; } }
Hiển thị biến property ra view.
app.component.html
<h2> App Component </h2> {{todayDate}} <p>Property: {{property}}</p> <app-new-cmp></app-new-cmp>
Gán MyserviceService.serviceproperty nó cho biến NewCmpComponent.property .
new-cmp.component.ts
import { Component, OnInit } from '@angular/core'; import { MyserviceService } from '../myservice.service'; @Component({ selector: 'app-new-cmp', templateUrl: './new-cmp.component.html', styleUrls: ['./new-cmp.component.css'] }) export class NewCmpComponent implements OnInit { newcomponent = "New Component"; todayDate: Date; property: string; constructor(private myservice: MyserviceService) { } ngOnInit() { this.todayDate = this.myservice.showTodayDate(); this.property = this.myservice.serviceproperty; } }
Hiển thị biến property ra view.
new-cmp.component.html
<p> {{newcomponent}} </p> <p> Today's Date : {{todayDate}} <br> Property: {{property}} </p>
Kết quả:
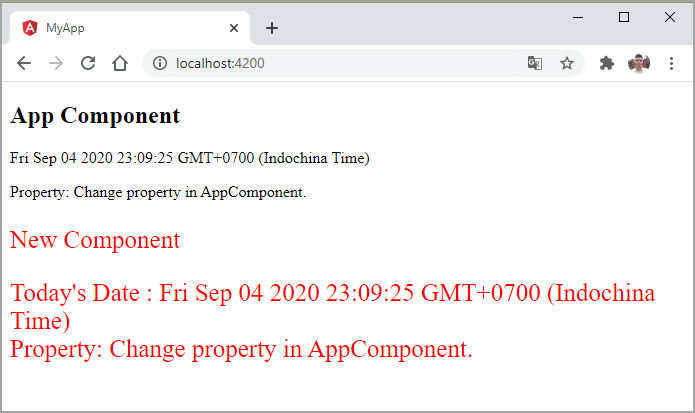