Ví dụ Ajax với Database
Đối tượng XMLHttpRequest trong AJAX Các công nghệ sử dụng Các công nghệ sử dụng trong ví dụ này: JDK 1.8 Apache Tomcat v8.5 MySQL 10.1.29-MariaDB (cài đặt xampp-win32-7.2.0-0-VC15-installer) Cấu trúc của project: Các bước để tạo ví dụ ajax với jsp ...
Các công nghệ sử dụng
Các công nghệ sử dụng trong ví dụ này:
- JDK 1.8
- Apache Tomcat v8.5
- MySQL 10.1.29-MariaDB (cài đặt xampp-win32-7.2.0-0-VC15-installer)
Cấu trúc của project:
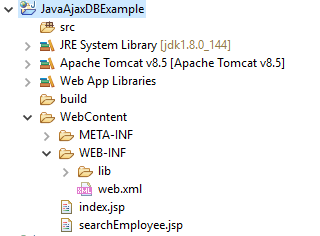
Các bước để tạo ví dụ ajax với jsp
Các bước để tạo ví dụ ajax với jsp:
- Tạo bảng testdb.EMPLOYEE.
- Tạo trang index.jsp tiếp nhận yêu cầu.
- Tạo trang tinhtong.jsp để xử lý yêu cầu.
- Cấu hình trang web mặc định (index) trong tệp web.xml
Tạo bảng testdb.EMPLOYEE
create table testdb.EMPLOYEE ( id INT NOT NULL auto_increment, first_name VARCHAR(20) default NULL, last_name VARCHAR(20) default NULL, salary INT default NULL, PRIMARY KEY (id) );
Tạo trang tiếp nhận yêu cầu
Trong trang này, chúng tôi đã tạo form nhận dữ liệu nhập từ người dùng. Khi người dùng nhấp vào nút Search, hàm sendInfo() được gọi. Ajax được sử dụng bên trong hàm này.
Chúng ta đã gọi hàm getInfo() bất cứ khi nào trạng thái sẵn sàng thay đổi. Nó ghi dữ liệu trả về vào trang web hiện tại một cách năng động bởi sự trợ giúp của thuộc tính innerHTML.
File: index.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Java Ajax Example</title> <script> var request; function sendInfo() { var empId = document.searchForm.employeeId.value; var url = "searchEmployee.jsp?id=" + empId; if (window.XMLHttpRequest) { request = new XMLHttpRequest(); } else if (window.ActiveXObject) { request = new ActiveXObject("Microsoft.XMLHTTP"); } try { request.onreadystatechange = getInfo; request.open("GET", url, true); request.send(); } catch (e) { alert("Unable to connect to server"); } } function getInfo() { if (request.readyState == 4) { var val = request.responseText; document.getElementById('result').innerHTML = val; } } </script> </head> <body> <h1>Ví dụ Java Ajax</h1> <form name="searchForm"> <input type="text" name="employeeId"> <input type="button" value="Search" onClick="sendInfo()"> </form> <span id="result"> </span> </body> </html>
Tạo trang bên máy chủ để xử lý yêu cầu
Trong trang jsp này, chúng ta tính tổng của 2 số được truyền qua url parameter.
File: tinhtong.jsp
<%@page import="java.sql.ResultSet"%> <%@page import="java.sql.PreparedStatement"%> <%@page import="java.sql.DriverManager"%> <%@page import="java.sql.Connection"%> <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Java Ajax Example</title> </head> <body> <% String idStr = request.getParameter("id"); if (idStr == null || idStr.trim().equals("")) { out.print("Please enter id"); } else { int id = Integer.parseInt(idStr); out.print(id); out.print("<br>"); try { Class.forName("com.mysql.jdbc.Driver"); Connection conn = DriverManager.getConnection( "jdbc:mysql://localhost:3306/testdb", "root", "1234567890"); PreparedStatement ps = conn.prepareStatement( "select * from employee where id=?"); ps.setInt(1, id); ResultSet rs = ps.executeQuery(); while (rs.next()) { out.print(rs.getInt(1) + " | " + rs.getString(2) + " " + rs.getString(3) ); } conn.close(); } catch (Exception e) { e.printStackTrace(); } } %> </body> </html>
Cấu hình trang web mặc định (index) trong tệp web.xml
File: web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" id="WebApp_ID" version="3.1"> <display-name>JavaAjaxDBExample</display-name> <welcome-file-list> <welcome-file>index.jsp</welcome-file> </welcome-file-list> </web-app>
Kết quả
Chạy ứng dụng và chúng ta có kết quả như sau:
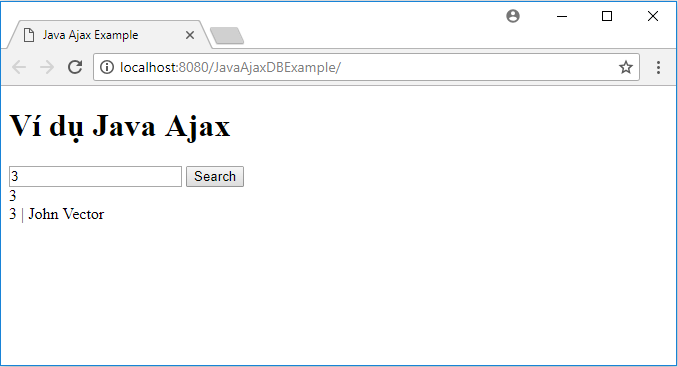