Xây dựng ứng dụng chat realtime bằng Laravel + Vuejs + Socket + Redis
Laravel giúp dễ dàng xây dựng các ứng dụng hiện đại với các tương tác thời gian thực bằng cách cung cấp một ‘event broadcasting system’ cho phép các nhà phát triển chia sẻ cùng tên sự kiện giữa server và ứng dụng JavaScript phía client. Trong bài này tôi sẽ chia sẻ làm ...
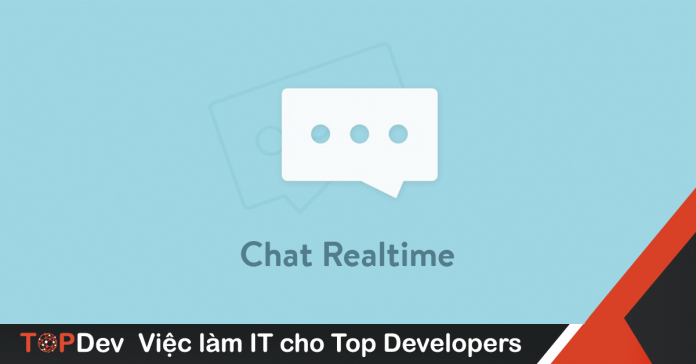
Laravel giúp dễ dàng xây dựng các ứng dụng hiện đại với các tương tác thời gian thực bằng cách cung cấp một ‘event broadcasting system’ cho phép các nhà phát triển chia sẻ cùng tên sự kiện giữa server và ứng dụng JavaScript phía client.
Trong bài này tôi sẽ chia sẻ làm như thế nào để build chat app bằng laravel, Socket.IO, Redis và Vuejs.
Có thể bạn quan tâm:
I. Cài đặt
1. Khởi tạo project
Bật Terminal lên, chúng ta khởi tạo project bằng lệnh:
1 2 3 |
Laravel new Chat |
hoặc:
1 2 3 |
composer create-project --prefer-dist laravel/laravel Chat` |
Sau khi tạo xong, chúng ta di chuyển vào trong project vừa tạo, rồi cài Redis như sau:
1 2 3 |
composer require predis/predis |
2. Setup Vuejs
Vì Laravel đã tích hợp sẵn Vuejs vào project của mình nên chỉ cần chạy:
1 2 3 |
npm install |
II. Thực hiện
Đầu tiên chúng ta tạo migration table messages:
1 2 3 4 5 6 7 8 |
Schema::create('messages', function (Blueprint $table) { $table->increments('id'); $table->text('message'); $table->integer('user_id')->unsigned(); $table->timestamps(); }); |
Rồi chạy lệnh:
1 2 3 |
php artisan migrate |
Sau khi chạy xong laravel sẽ có table messages và table users.
Để chat được, user cần phải đăng nhập vào hệ thống, ta chỉ cần chạy lệnh:
1 2 3 |
php artisan make:auth |
Sau đó chúng ta thêm vào router như sau:
1 2 3 4 5 6 7 8 9 10 |
//lấy tất cả các messages, và sẽ có form để chat Route::get('messages', 'MessageController@index'); //insert chat content vào trong database Route::post('messages', 'MessageController@store'); //lấy ra user hiện tại Route::get('current-user', 'UserController@currentUser'); |
Tiếp theo chúng ta tạo ra model, controller Message và User:
1 2 3 |
php artisan make:model Messsage -c |
Câu lệnh trên sẽ tạo ra Model Message và MessageController
Tạo ra UserController:
1 2 3 |
php artisan make:controller UserController |
Model của Message như sau:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
<?php namespace App; use IlluminateDatabaseEloquentModel; class Message extends Model { protected $fillable = ['message', 'user_id']; public function user() { return $this->belongsTo('AppUser'); } } |
Model của User sẽ như sau:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 |
<?php namespace App; use IlluminateNotificationsNotifiable; use IlluminateContractsAuthMustVerifyEmail; use IlluminateFoundationAuthUser as Authenticatable; class User extends Authenticatable { use Notifiable; /** * The attributes that are mass assignable. * * @var array */ protected $fillable = [ 'name', 'email', 'password', ]; /** * The attributes that should be hidden for arrays. * * @var array */ protected $hidden = [ 'password', 'remember_token', ]; public function message() { return $this->hasMany('AppMessage'); } } |
Trong MessageController sẽ như sau:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
<?php namespace AppHttpControllers; use IlluminateHttpRequest; use AppMessage; class MessageController extends Controller { public function index() { if ($messages = Redis::get('messages.all')) { return json_decode($messages); } $messages = AppMessage::with('user')->get(); Redis::set('messages.all', $messages); return view('welcome'); } public function store() { $user = Auth::user(); $message = AppMessage::create(['message'=> request()->get('message'), 'user_id' => $user->id]); broadcast(new MessagePosted($message, $user))->toOthers(); return $message; } } |
Sau đó chúng ta cần tạo một Event MessagePosted bằng lệnh:
1 2 3 |
php artisan make:event MessagePosted |
Trong MessagePosted có nội dung như sau:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 |
<?php namespace AppEvents; use IlluminateBroadcastingChannel; use IlluminateQueueSerializesModels; use IlluminateBroadcastingPrivateChannel; use IlluminateBroadcastingPresenceChannel; use IlluminateFoundationEventsDispatchable; use IlluminateBroadcastingInteractsWithSockets; use IlluminateContractsBroadcastingShouldBroadcast; use AppMessage; use AppUser; class MessagePosted implements ShouldBroadcast { use Dispatchable, InteractsWithSockets, SerializesModels; public $message; public $user; /** * Create a new event instance. * * @return void */ public function __construct(Message $message, User $user) { $this->message = $message; $this->user = $user; } /** * Get the channels the event should broadcast on. * * @return IlluminateBroadcastingChannel|array */ public function broadcastOn() { return new Channel('chatroom'); } } |
Và đừng quên vào config/app.js phần providers bỏ comment dòng này đi nhé
1 2 3 |
AppProvidersBroadcastServiceProvider::class, |
Ta cần update lại file .env
1 2 3 4 5 6 7 |
BROADCAST_DRIVER=redis CACHE_DRIVER=redis SESSION_DRIVER=redis SESSION_LIFETIME=120 QUEUE_DRIVER=redis |
Phần backend như thế coi như xong. Tiếp theo chúng ta sẽ làm việc với Vuejs Trước tiên ta cần cài VueX và Axios vào trong project của mình:
npm install vuex –save
npm install axios –save
Chúng ta tạo ra 2 component là: ChatLayout và ChatItem trong resources/js/components.
Video: Deep learning với PyTorch và những ứng dụng
Trong file ChatLayout có nội dung như sau:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101
Có thể bạn quan tâm
0
|