MVC trong JSP
Học servlet MVC là viết tắt của Model View Controller là một mẫu thiết kế phần mềm được chia thành 3 phần riêng biệt đó là xử lý nghiệp vụ, xử lý giao diện và dữ liệu. Ví dụ về MVC trong JSP Trong ví dụ này chúng ta sử dụng servlet như một controller, jsp như một ...
MVC là viết tắt của Model View Controller là một mẫu thiết kế phần mềm được chia thành 3 phần riêng biệt đó là xử lý nghiệp vụ, xử lý giao diện và dữ liệu.
Ví dụ về MVC trong JSP
Trong ví dụ này chúng ta sử dụng servlet như một controller, jsp như một thành phần view và lớp java bean như một model.
Cấu trúc project:
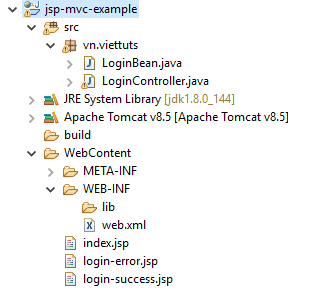
Trong đó:
- index.jsp là trang thu thập thông tin người dùng.
- ControllerServlet.java một servlet được cài đặt như một controller.
- login-success.jsp và login-error.jsp là các view.
- web.xml là file mapping servlet.
File: LoginBean.java
package vn.viettuts; public class LoginBean { private String name, password; public String getName() { return name; } public void setName(String name) { this.name = name; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } public boolean validate() { if ("admin".equals(password)) { return true; } else { return false; } } }
File: LoginController.java
package vn.viettuts; import java.io.IOException; import java.io.PrintWriter; import javax.servlet.RequestDispatcher; import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; public class LoginController extends HttpServlet { protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("text/html"); PrintWriter out = response.getWriter(); String name = request.getParameter("name"); String password = request.getParameter("password"); LoginBean bean = new LoginBean(); bean.setName(name); bean.setPassword(password); request.setAttribute("bean", bean); boolean status = bean.validate(); if (status) { RequestDispatcher rd = request .getRequestDispatcher("login-success.jsp"); rd.forward(request, response); } else { RequestDispatcher rd = request .getRequestDispatcher("login-error.jsp"); rd.forward(request, response); } } @Override protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { doPost(req, resp); } }
File: web.xml
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" id="WebApp_ID" version="3.1"> <display-name>jsp-mvc-example</display-name> <welcome-file-list> <welcome-file>index.jsp</welcome-file> </welcome-file-list> <servlet> <servlet-name>LoginController</servlet-name> <servlet-class>vn.viettuts.LoginController</servlet-class> </servlet> <servlet-mapping> <servlet-name>LoginController</servlet-name> <url-pattern>/login</url-pattern> </servlet-mapping> </web-app>
File: index.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Vi du MVC trong JSP</title> </head> <body> <form action="login" method="post"> Username: <input type="text" name="name"><br> Password:<input type="password" name="password"><br> <input type="submit" value="login"> </form> </body> </html>
File: login-success.jsp
<%@page import="vn.viettuts.LoginBean"%> <%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Login Success</title> </head> <body> <p>You are successfully logged in!</p> <% LoginBean bean = (LoginBean) request.getAttribute("bean"); out.print("Welcome, " + bean.getName()); %> </body> </html>
File: login-error.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Login Error</title> </head> <body> <p>username or password is incorrect!</p> <%@ include file="index.jsp"%> </body> </html>
Run ứng dụng
Hiển thị ban đầu:
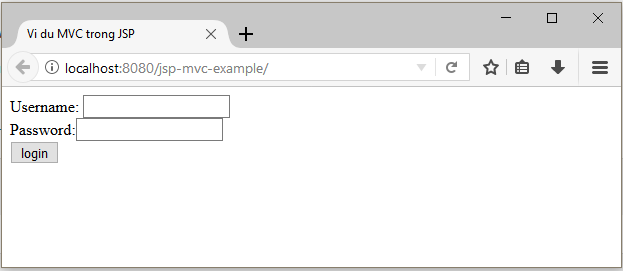
Nhập Username=Lucky và Password=”admin”:
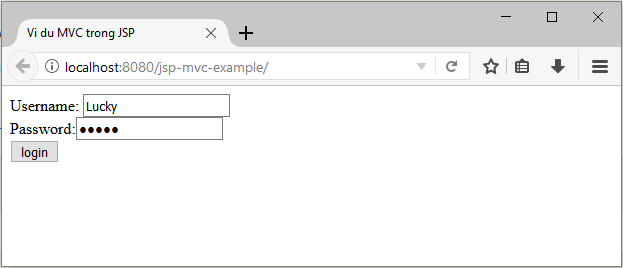
Click Login:
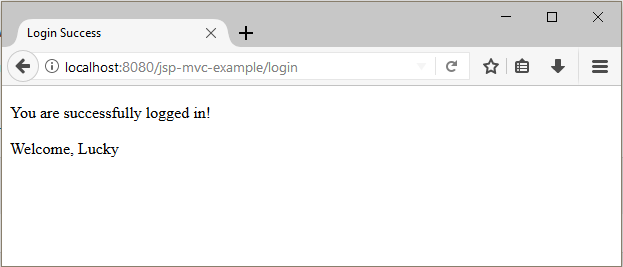