Ví dụ login trong Struts2
Cấu hình Struts2 Các công nghệ sử dụng trong ví dụ này eclipse mar2 apache-tomcat-7.0.75 struts-2.3.3 jdk 1.8 Maven 3.5 Cấu trúc của project “struts2-login-example” Tạo project “struts2-login-example” Tạo project bằng cách ...
Các công nghệ sử dụng trong ví dụ này
- eclipse mar2
- apache-tomcat-7.0.75
- struts-2.3.3
- jdk 1.8
- Maven 3.5
Cấu trúc của project “struts2-login-example”
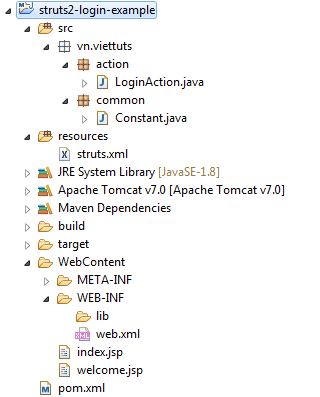
Tạo project “struts2-login-example”
Tạo project bằng cách chọn File–>New–>Dynamic Web Project.
Add struts2 library
Cách 1: Sao chép các tập tin sau từ struts2 thư mục lib C:libstruts-2.3.3lib vào thư mục WEB-INFlib của project. Để thực hiện việc này, bạn chỉ cần kéo và thả tất cả các tệp sau vào thư mục WEB-INFlib.
- asm-xy.jar
- asm-commons-xy.jar
- asm-tree-xy.jar
- commons-fileupload-xyz.jar
- commons-langxy.jar
- commons-io-xy.jar
- freemarker-xyz.jar
- javassist-.xyz.GA
- ognl-xyz.jar
- struts2-core-xyz.jar
- xwork-core.xyz.jar
Cách 2: convert project sang maven project rồi add dependency vào file pom.xml như sau:
<dependencies> <dependency> <groupId>org.apache.struts</groupId> <artifactId>struts2-core</artifactId> <version>2.3.3</version> </dependency> </dependencies>
Cấu hình web.xml
Sửa file web.xml như sau:
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <display-name>struts2-login-example</display-name> <welcome-file-list> <welcome-file>index.jsp</welcome-file> </welcome-file-list> <filter> <filter-name>struts2</filter-name> <filter-class> org.apache.struts2.dispatcher.FilterDispatcher </filter-class> </filter> <filter-mapping> <filter-name>struts2</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> </web-app>
Tạo file struts.xml
Tạo file strtus.xml tại thư mục resources của project:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.0//EN" "http://struts.apache.org/dtds/struts-2.0.dtd"> <struts> <constant name="struts.devMode" value="true" /> <package name="default" namespace="/" extends="struts-default"> <action name="login" class="vn.viettuts.action.LoginAction" method="login"> <result name="success">/welcome.jsp</result> <result name="error">/index.jsp</result> </action> </package> </struts>
Tạo trang chứa login form
Tạo file index.jsp tại thư mục WebContent của project. Trong file này chúng ta định nghĩa một form gồm 2 thuộc tính có tên userName và password.
File: index.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8" %> <%@ taglib prefix="s" uri="/struts-tags"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Struts2 - Login Example</title> <style type="text/css"> .error-msg { color: red; } </style> </head> <body> <s:form id="loginForm" class="loginForm" theme="css_xhtml"> <div class="error-msg"> <s:actionerror /> </div> <table class="login"> <tbody> <tr> <td><s:label value="UserName:" for="userName" /></td> <td><s:textfield name="userName" id="userName" /></td> </tr> <tr> <td><s:label value="Password:" for="password" /></td> <td><s:password name="password" id="password" /></td> </tr> <tr> <td><s:submit name="login" value="Login" onclick="this.form.action='login'" /> </td> </tr> </tbody> </table> </s:form> </body> </html>
Tạo trang welcome.jsp
Tạo file welcome.jsp tại thư mục WebContent của project. Trang này hiện thị tên userName được nhập từ trang index.jsp.
File: welcome.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"%> <%@ taglib prefix="s" uri="/struts-tags"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>Struts2 - Welcome</title> </head> <body> Welcome, <s:property value="userName" /> </body> </html>
Tạo action
Tạo action login LoginAction.java tại package vn.viettuts.action của project.
File: LoginAction.java
package vn.viettuts.action; import com.opensymphony.xwork2.ActionSupport; import vn.viettuts.common.Constant; public class LoginAction extends ActionSupport { private String userName; private String password; /** * login method * * @return success or error */ public String login() { if (!(Constant.USERNAME.equals(userName) && Constant.PASSWORD.equals(password))) { addActionError(Constant.INVALID_ACCOUNT_MSG); return ERROR; } return SUCCESS; } // define setter & setter public String getUserName() { return userName; } public void setUserName(String userName) { this.userName = userName; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } }
Tạo lớp Constant.java
Tạo lớp Constant.java tại package vn.viettuts.common của project. Vì đây là ứng dụng nhỏ chưa có kết nối đến database nên chúng ta định nghĩa các username, password, và error mesage giả định trong lớp Constant.java.
File: Constant.java
package vn.viettuts.common; public class Constant { public static final String USERNAME = "admin"; public static final String PASSWORD = "123456"; public static final String INVALID_ACCOUNT_MSG = "username or password is incorrect!"; }
Thực thi project “struts2-login-example”
Hiển thị ban đầu:
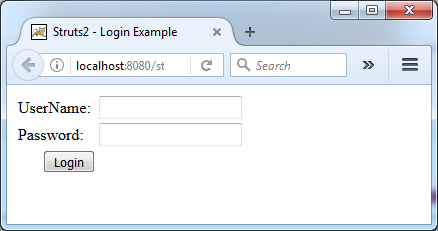
Login fail:
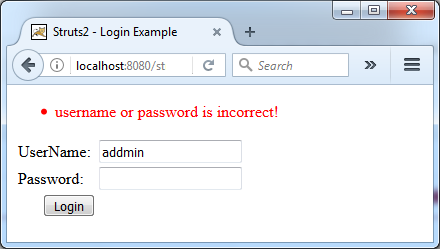
Login success:
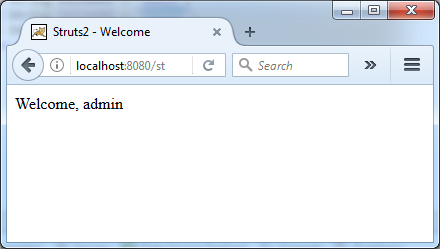
Download Source Code
Download source code + thư viện:
Download source code maven: