Pipe trong Angular 7
Directive trong Angular 7 Nội dung chính Giới thiệu Pipe trong Angular 7 Ví dụ sử dụng Pipe biến chuỗi đã cho thành chữ hoa, chữ thường Một số pipe có sẵn trong Angular Làm thế nào để tạo pipe tủy chỉnh trong Angular? Giới thiệu Pipe trong Angular 7 ...
Nội dung chính
- Giới thiệu Pipe trong Angular 7
- Ví dụ sử dụng Pipe biến chuỗi đã cho thành chữ hoa, chữ thường
- Một số pipe có sẵn trong Angular
- Làm thế nào để tạo pipe tủy chỉnh trong Angular?
Giới thiệu Pipe trong Angular 7
Bài này, chúng ta sẽ tìm hiểu về Pipe trong Angular 7. Pipe trước đó được gọi là Filter trong Angular1 và được gọi là Pipe từ Angular2 trở đi.
Ký tự | được sử dụng để biến đổi dữ liệu. Sau đây là một ví dụ biến đổi chuỗi đã cho thành chuỗi chữ thường.
{{ Welcome to Angular 7 | lowercase}}
Ví dụ sử dụng Pipe biến chuỗi đã cho thành chữ hoa, chữ thường
Trong file app.component.ts, chúng ta định nghĩa biến title như sau:
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 7 Project!'; }
Thêm đoạn code sau vào file app.component.html
<b>{{title | uppercase}}</b><br/> <b>{{title | lowercase}}</b>
File app.component.html có nội dung như sau:
<div style="text-align:center"> <h1> <b>{{title | uppercase}}</b><br/> <b>{{title | lowercase}}</b> </h1> </div> <router-outlet></router-outlet>
Kết quả:
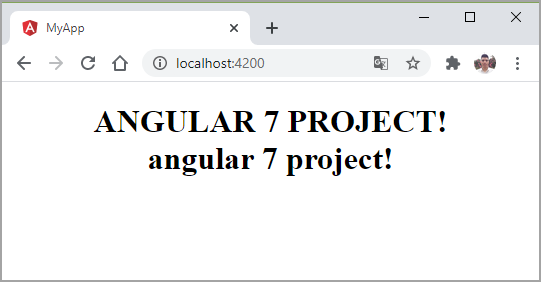
Một số pipe có sẵn trong Angular
- Lowercase pipe
- Uppercase pipe
- Date pipe
- Currency pipe
- Json pipe
- Percent pipe
- Decimal pipe
- Slice pipe
Ở trên chúng ta đã có một ví dụ về pipe biến đổi chữ hoa, chữ thường. Bây giờ chúng ta sẽ tìm hiểu các pipe khác.
Định nghĩa các biến trong file app.component.ts :
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 7 Project!'; todayDate = new Date(); jsonData = {name:'Vinh', age:'18', address:{huyen:'Me Linh', tp:'Ha Noi'}}; months = ["Jan", "Feb", "Mar", "April", "May", "Jun", "July", "Aug", "Sept", "Oct", "Nov", "Dec"]; }
Chúng ta sẽ sử dụng pipe trong file app.component.html như sau:
<div style="text-align:center"> <h1> <b>{{title | uppercase}}</b> </h1> </div> <div style = "awidth:100%;"> <div style = "awidth:40%;float:left;border:solid 1px black;"> <h1>Lowercase Pipe</h1> <b>{{title | lowercase}}</b> <h1>Currency Pipe</h1> <b>{{6589.23 | currency:"USD"}}</b> <br/> <b>{{6589.23 | currency:"USD":true}}</b> // Boolean true được sử dụng để lấy ký hiệu của tiền tệ. <h1>Date pipe</h1> <b>{{todayDate | date:'dd/MM/yyyy'}}</b> <br/> <b>{{todaydate | date:'shortTime'}}</b> <h1>Decimal Pipe</h1> <b>{{ 454.78787814 | number: '3.4-4' }}</b> // 3 số trước dấu phẩy, 4 -4 số chữ số được hiển thị sau dấu phẩy. </div> <div style = "awidth:40%;float:left;border:solid 1px black;"> <h1>Json Pipe</h1> <b>{{ jsonData | json }}</b> <h1>Percent Pipe</h1> <b>{{00.54565 | percent}}</b> <h1>Slice Pipe</h1> <b>{{months | slice:2:6}}</b> // here 2 and 6 refers to the start and the end index </div> </div> <router-outlet></router-outlet>
Kết quả:
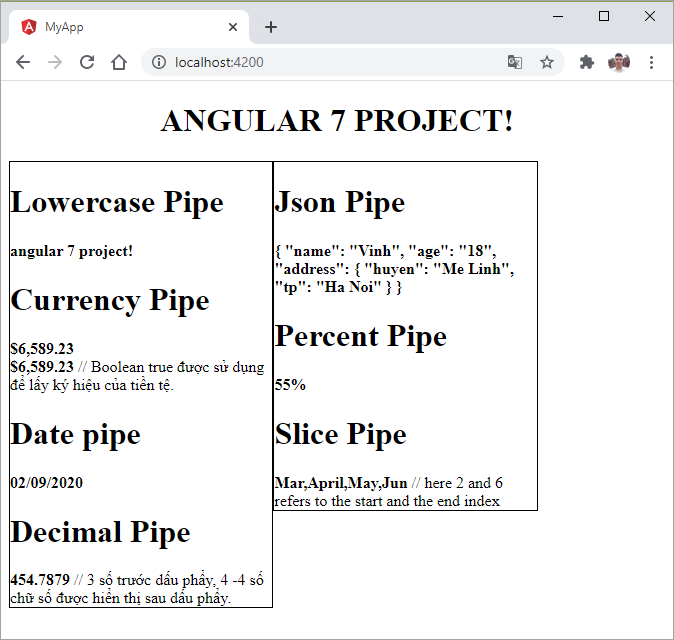
Làm thế nào để tạo pipe tủy chỉnh trong Angular?
Để tạo một pipe tùy chỉnh, chúng ta cần phải tạo một file .ts mới.
Ví dụ 1: Tạo pipe tính căn bậc 2. Ở đây, chúng ta sẽ tạo pipe tùy chỉnh sqrt để tính căn bậc 2 của một số. Tạo file app.sqrt.ts bên trong thư mục src/app như sau:
import {Pipe, PipeTransform} from '@angular/core'; @Pipe ({ name : 'sqrt' }) export class SqrtPipe implements PipeTransform { transform(val : number) : number { return Math.sqrt(val); } }
Để tạo một pipe tùy chỉnh, chúng ta phải import Pipe và PipeTransform từ Angular/core. Trong chỉ thị @Pipe, bạn phải đặt tên cho pipe, tên này sẽ được sử dụng trong tệp .html.
Tiếp theo, bạn phải tạo lớp và tên lớp là SqrtPipe. Lớp này sẽ triển khai lớp PipeTransform.
Phương thức biến đổi được định nghĩa trong lớp sẽ nhận đối số là số và sẽ trả về số sau khi lấy căn bậc hai.
Vì lớp SqrtPipe được tạo mới nên bạn cần khai báo nó trong module app.module.ts như sau:
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AppRoutingModule } from './app-routing.module'; import { AppComponent } from './app.component'; import { NewCmpComponent } from './new-cmp/new-cmp.component'; import { AddTextDirective } from './add-text.directive'; import { SqrtPipe } from './app.sqrt'; @NgModule({ declarations: [ AppComponent, NewCmpComponent, AddTextDirective, SqrtPipe ], imports: [ BrowserModule, AppRoutingModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
Bây giờ chúng ta sẽ tạo ra lệnh gọi được thực hiện tới pipe sqrt trong file app.component.html.
<div style="text-align:center"> <h1> <b>{{title | uppercase}}</b> </h1> </div> <h2>Custom Pipe</h2> <b>Can bac 2 cua 25 la: {{25 | sqrt}}</b> <br/> <b>Can bac 2 cua 729 la: {{729 | sqrt}}</b> <router-outlet></router-outlet>
Kết quả:
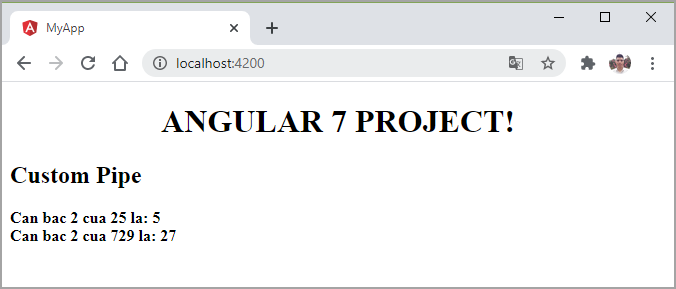
Ví dụ 2: Tạo pipe tính bình phương của một số.
Bây giờ chúng ta sẽ tạo pipe để tính bình phương của một số.
Tạo pipe square trong file app.sqrt.ts để tính bình phương của một số:
import {Pipe, PipeTransform} from '@angular/core'; @Pipe ({ name : 'sqrt' }) export class SqrtPipe implements PipeTransform { transform(val : number) : number { return Math.sqrt(val); } } @Pipe ({ name : 'square' }) export class SquarePipe implements PipeTransform { transform(val : number) : number { return val * val; } }
Cập nhật file app.module.ts, khai báo lớp SquarePipe trong app module:
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AppRoutingModule } from './app-routing.module'; import { AppComponent } from './app.component'; import { NewCmpComponent } from './new-cmp/new-cmp.component'; import { AddTextDirective } from './add-text.directive'; import { SqrtPipe, SquarePipe } from './app.sqrt'; @NgModule({ declarations: [ AppComponent, NewCmpComponent, AddTextDirective, SqrtPipe, SquarePipe ], imports: [ BrowserModule, AppRoutingModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
Sử dụng pipe trong template app.component.ts
<div style="text-align:center"> <h1> <b>{{title | uppercase}}</b> </h1> </div> <h2>Custom Pipe</h2> <b>Can bac 2 cua 25 la: {{25 | sqrt}}</b> <br/> <b>Can bac 2 cua 729 la: {{729 | sqrt}}</b> <h2> Square Pipe </h2> <b>Binh phuong cua 5 la: {{5 | square}}</b> <br/> <b>Binh phuong cua 9 la: {{9 | square}}</b> <router-outlet></router-outlet>
Kết quả:
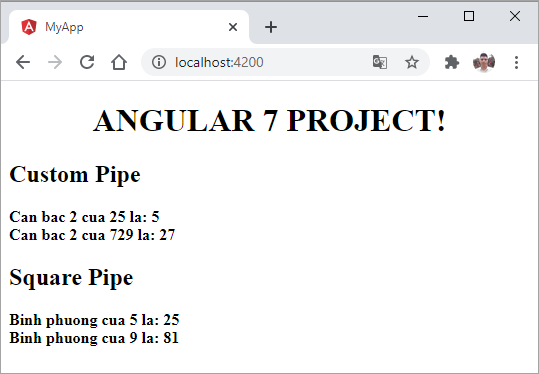